How to Use a Head Manager in a Vue Project
"4 years ago"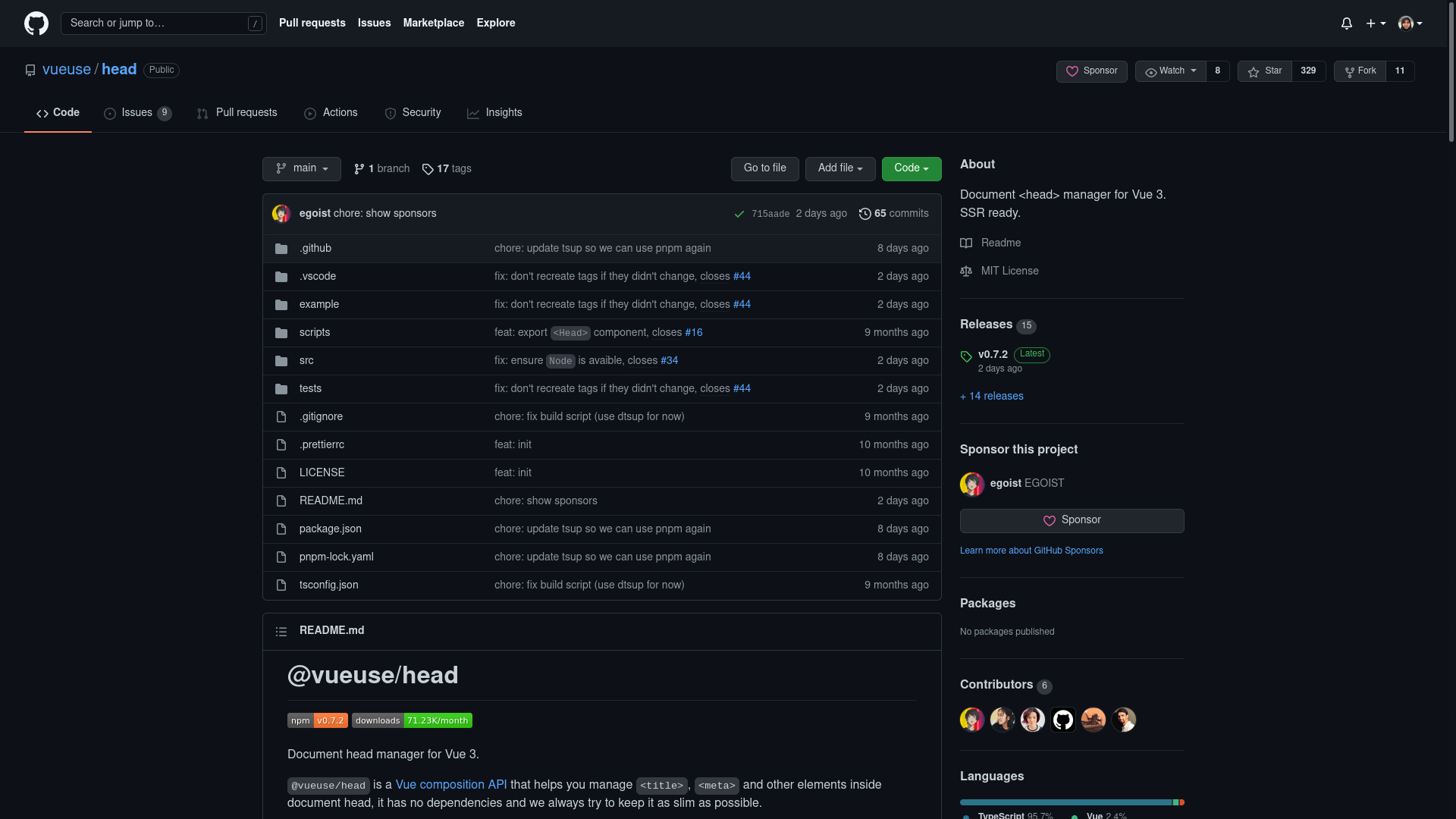
@vueuse/head is a document <head>
manager for Vue 3.
Installation
Create a Vue project and install the packages.
- @vueuse/head
- unplugin-vue-components optional - I include it for the convenience of components auto import
pnpm create vite vue-3-head -- --template vue
cd vue-3-head
pnpm i @vueuse/head
pnpm i -D unplugin-vue-components
pnpm create vite vue-3-head -- --template vue
cd vue-3-head
pnpm i @vueuse/head
pnpm i -D unplugin-vue-components
Configuration
- Register the plugin in
src/main.js
.
import { createApp } from "vue"
import { createHead } from "@vueuse/head"
import App from "./App.vue"
const app = createApp(App)
const head = createHead()
app.use(head).mount("#app")
import { createApp } from "vue"
import { createHead } from "@vueuse/head"
import App from "./App.vue"
const app = createApp(App)
const head = createHead()
app.use(head).mount("#app")
- Register the other plugin in
vite.config.js
.
import { defineConfig } from "vite"
import Vue from "@vitejs/plugin-vue"
import Components from "unplugin-vue-components/vite"
export default defineConfig({
plugins: [Vue(), Components()]
})
import { defineConfig } from "vite"
import Vue from "@vitejs/plugin-vue"
import Components from "unplugin-vue-components/vite"
export default defineConfig({
plugins: [Vue(), Components()]
})
Usage
- Create a component in
src/components
, e.g.TheHead.vue
. Here I set the<Head>
to use thetitle
anddescription
passed to this component.
<template>
<Head>
<title>{{ title }}</title>
<meta name="description" :content="description" />
</Head>
</template>
<script setup>
import { Head } from "@vueuse/head"
const props = defineProps({
title: {
type: String,
default: ""
},
description: {
type: String,
default: ""
}
})
</script>
<template>
<Head>
<title>{{ title }}</title>
<meta name="description" :content="description" />
</Head>
</template>
<script setup>
import { Head } from "@vueuse/head"
const props = defineProps({
title: {
type: String,
default: ""
},
description: {
type: String,
default: ""
}
})
</script>
Here is the syntax for other elements, taken from the repo example.
<template>
<Head>
<html lang="en"></html>
<body class="body"></body>
<component is="style">
body { background: lightgreen; } {{ style }}
</component>
</Head>
</template>
<script setup>
import { Head } from "@vueuse/head"
const style = `button { color: red; }`
</script>
<template>
<Head>
<html lang="en"></html>
<body class="body"></body>
<component is="style">
body { background: lightgreen; } {{ style }}
</component>
</Head>
</template>
<script setup>
import { Head } from "@vueuse/head"
const style = `button { color: red; }`
</script>
- Use the component wherever you need. With
unplugin-vue-components
installed, we do not need to import the component.
<template>
<TheHead
title="Yasmin ZY"
description="Personal website of Yasmin Zulfati Yusrina. I create tutorials about Vue.js." />
</template
<template>
<TheHead
title="Yasmin ZY"
description="Personal website of Yasmin Zulfati Yusrina. I create tutorials about Vue.js." />
</template